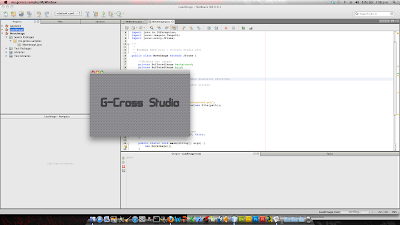
Hi, this is one simple code for load and show an image inside a JFrame, using a BufferedImage to load image data from a specific local path (could be a URL if you prefer).
Steps:
1.- Create a BufferedImage object.
2.- Read the image file for a specific path
3.- Draw the image in the screen using "paint()" method.
That's it!
package mx.gcross.samples;
import java.awt.Graphics;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.JFrame;
/**
*
* @author Rene Cruz - G-Cross Studio 2011
*/
public class MyWindow extends JFrame {
//Object for image
private BufferedImage background;
public MyWindow() {
setSize(480, 320); //set window dimension 480x320px
loadImages();
setVisible(true); //make window visible
}
public void loadImages() {
try {
//path for image file
String path = "images/background.png";
background = ImageIO.read(new File(path));
} catch (IOException ex) {
ex.printStackTrace();
}
}
@Override
public void paint(Graphics g) {
super.paint(g);
//draw the bufferedImage object
g.drawImage(background, 0, 0, this);
}
public static void main(String[] args) {
new MyWindow();
}
}
Test the code and comment, thanks!.
If you prefer the complete code source and resources in a Netbeans project, Download It Here!
No hay comentarios:
Publicar un comentario